What Are Design Patterns?
Arriving at designs that are specific to the problem at hand but general enough to address future problems and requirements is hard. New designers tend to be overwhelmed by the options available. Expert designers do not solve every problem from first principles; they reuse design patterns.
What is a design pattern? A collection of choices on how to go about solving a problem when designing systems. Sample problems: state synchronization, ownership relations, etc.
A design pattern has 4 elements:
- Pattern name: succinctly describes the design problem, its solutions, and its consequences.
- Problem: describes when to apply the pattern, e.g., how to represent algorithms as objects.
- Solution: describes the elements that make up the design, their relationships, responsibilities, and collaborations.
- Consequences: the results and tradeoffs of applying the pattern, e.g., space/time, language implementation issues, extensibility, etc.
Design patterns are not about designs such as hash tables that can be encoded in classes and reused as-is. Nor are they complex, domain-specific designs for an entire application or subsystem. Instead, they are descriptions of communicating objects and classes that are customized to solve a general design problem in a particular context.
Why use a design pattern?
- Helps you choose design alternatives that make a system reusable.
- Improve the documentation and maintenance of existing systems by adding explicit specifications of class and object interactions, and their underlying intent.
With the explicit goal of reusability, keep an eye out when we trade off some other desirable quality, like computational efficiency.
While experienced devs use design patterns exclusively, there is no good recording of the various design patterns used. aims to present a catalog of design patterns, and with a consistent format: name(s), classification, intent, motivation, applicability, graphical representation, participating classes/objects and their responsibilities, collaborations between participants, consequences, implementation notes, sample code, known uses, related patterns.
The choice of programming language is important because the language features determine what can and cannot be implemented easily. For example, CLOS has multi-methods, which lessen the need for a pattern such as Visitor.
The Model/View/Controller (MVC) triad of classes used to build UI in Smalltalk-80 helps illustrate various design patterns:
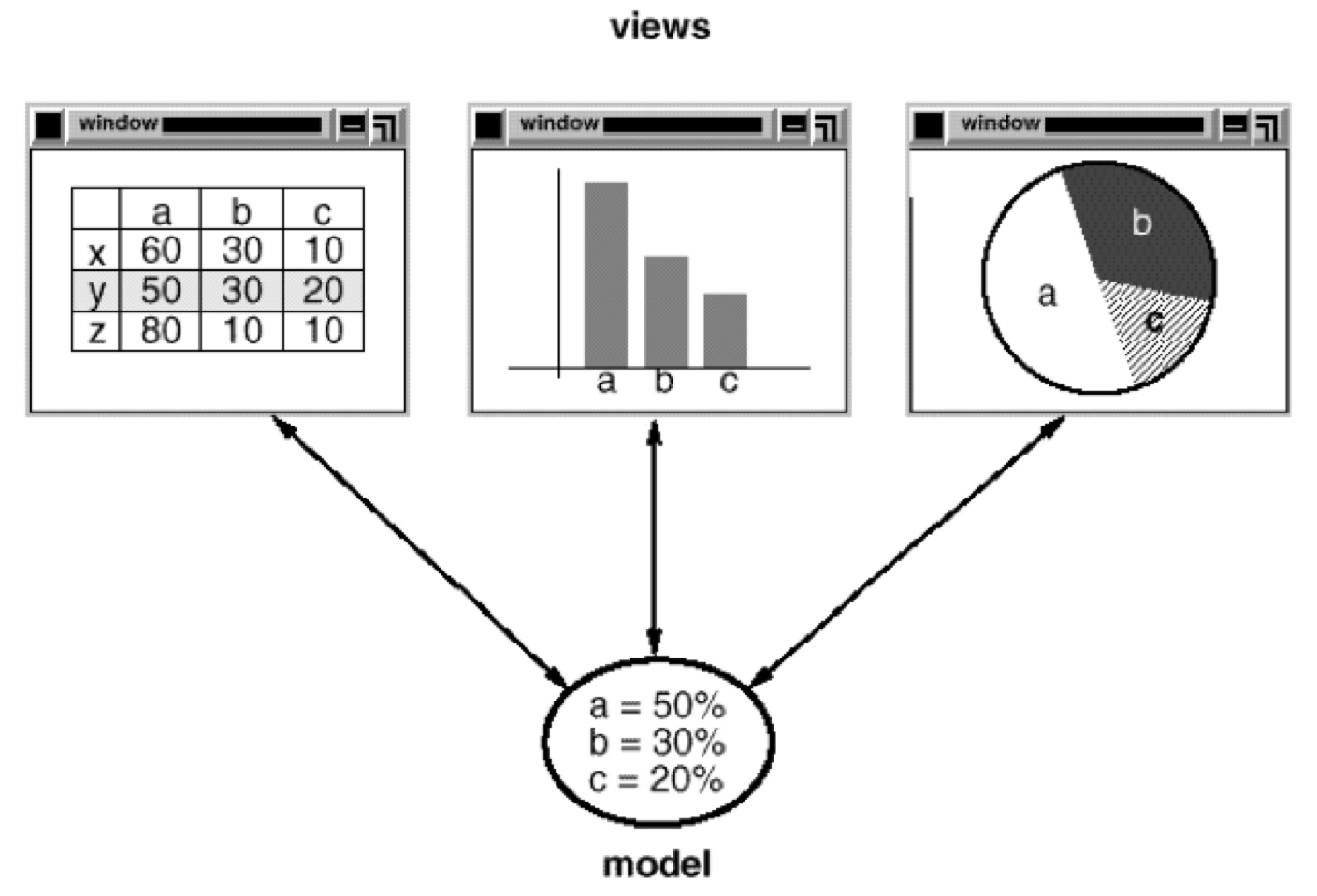
MVC diagram. Credits: GangOfFour1994-Ch1
- MVC has a design that decouples views from models. The more general problem is decoupling objects so that changes to one can affect any number of others without requiring the changed object to know the details of the others. The general design is described by the Observer design pattern.
- In MVC, a view can be composed of other views. The more general problem is grouping objects and treating the group like an individual object. The general design is described by the Composite design pattern.
- MVC lets one change how a view responds to user input without changing the visual representation, by encapsulating the response mechanism in a Controller object, e.g., a disabled view is given a controller that ignores input events. This View-Controller relationship is an example of the Strategy design pattern.
References
- Design Patterns. Ch 1. Introduction. Erich Gamma; Richard Helm; Ralph Johnson; John Vlissides. Oct 21, 1994.
I’ve also encountered the “you aren’t gonna need it” (YAGNI) school of thought that aims to minimize writing code that anticipates too far into the future, as such guesses usually don’t pan out. Design patterns seem like they exist somewhere between designing for now, and designing for a possible future. A design pattern solves a specific problem being encountered now. And even within a design pattern, one could still adhere to YAGNI, and add pieces when needed. When it does come to the point where the design needs more features, at least the design pattern provides a mental framework that is consistent with the initial design of the system.